iOS
https://www.raywenderlich.com/117609/sketch-indie-developers
Once you have gone through the basics with the iOS Apprentice, you may wish to brush up on these core concepts that every iOS developer should know.
These tutorials will help you understand the key views and view
controllers that you will use in your day-to-day life as an iOS
developer.
Learn how to draw in your app - and make things move!
Want to add a map into your app?
In these tutorials, you'll learn how to do this - along with customizing their look, and getting notification when your user's device enters or leaves certain regions.
Almost every app needs to save and load data on the iPhone - and
there are many different ways to do so. In these tutorials, you can get
hands-on experience with many of the most common methods.
You can take your app to the next level by integrating with a
server-back end or allowing networking between devices. These tutorials
show you how!
It's bad practice to perform long-running operations on your main
thread - it's better to use background threads. But that can get tricky
if you're not careful!
In these tutorials, you'll learn how to use concurrency the right way.
watchOS is the platform for Apple Watch. Learn how to get started building watch apps with these tutorials!
There are tons of other APIs in iOS - here are some tutorials on some of the most important ones!
There's more to iOS development than just learning APIs - as with any
form of development, you also need good software engineering practices.
Here are a few tutorials on some of those - from an iOS-specific perspective!
Apple isn't the only company making great APIs you'll want to use in your apps.
Here are some tutorials on some of the key tools and libraries you'll want to know about.
Apps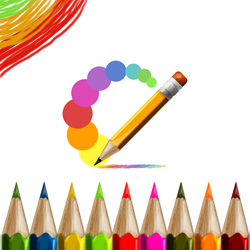
Core Concepts
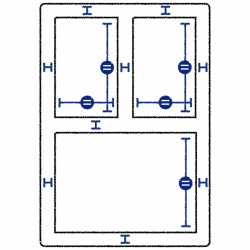
- Storyboards Tutorial in iOS 9: Part 1
- Storyboards Tutorial in iOS 9: Part 2
- Auto Layout Tutorial in iOS 9 Part 1: Getting Started
- Auto Layout Tutorial in iOS 9 Part 2: Constraints
- How To Secure iOS User Data: The Keychain and Touch ID
- iOS Unit Testing and UI Testing Tutorial
- Core Text Tutorial for iOS: Making a Magazine App
- UIAppearance Tutorial: Getting Started
- UISearchController Tutorial: Getting Started
- Top 10 Most Popular iOS Tutorials on raywenderlich.com
- Design Patterns on iOS using Swift – Part 1/2
- Design Patterns on iOS using Swift – Part 2/2
Views and View Controllers
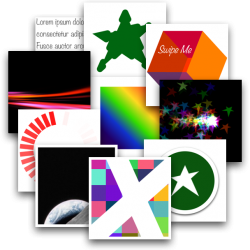
- UIVisualEffectView Tutorial: Getting Started
- CALayer Tutorial: Getting Started
- UISplitViewController Tutorial: Getting Started
- View Debugging in Xcode 6
- UICollectionView Custom Layout Tutorial: Pinterest
- Auto Layout Visual Format Language Tutorial
- Creating Custom UIViewController Transitions
- iOS 9 Storyboards Tutorial: What’s New in Storyboards?
- State Restoration Tutorial: Getting Started
- Easier Auto Layout: Coding Constraints in iOS 9
- Self-sizing Table View Cells
- UICollectionView Tutorial: Getting Started
- UICollectionView Tutorial: Reusable Views, Selection, and Reordering
- UIPresentationController Tutorial: Getting Started
- Custom UICollectionViewLayout Tutorial With Parallax
- UIScrollView Tutorial: Getting Started
- UIStackView Tutorial: Introducing Stack Views
- Adaptive Layout Tutorial in iOS 11: Getting Started
Graphics & Animation APIs
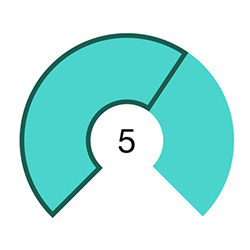
- UIKit Dynamics Tutorial: Getting Started
- Core Image Tutorial: Getting Started
- Core Graphics Tutorial Part 1: Getting Started
- Core Graphics Tutorial Part 2: Gradients and Contexts
- Core Graphics Tutorial Part 3: Patterns and Playgrounds
- UIKit Dynamics and Swift Tutorial: Tossing Views
- AsyncDisplayKit Tutorial: Node Hierarchies
- UIAppearance Tutorial: Getting Started
- iOS Animation Tutorial: Introduction to Easy Animation
- Apple Pencil Tutorial: Getting Started
- iOS Animation Tutorial: Getting Started
- iOS Animation Tutorial: Custom View Controller Presentation Transitions
Map & Location APIs
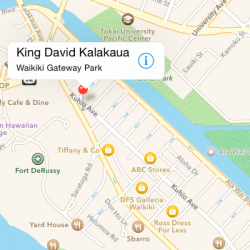
In these tutorials, you'll learn how to do this - along with customizing their look, and getting notification when your user's device enters or leaves certain regions.
- MapKit Tutorial: Overlay Views
- MapKit Tutorial: Getting Started
- Core Location Tutorial: Geofencing
- Routing with MapKit and Core Location
- Geofencing Tutorial with Core Location
- Augmented Reality iOS Tutorial: Location Based
- How To Make An App Like Pokemon Go
- iBeacon Tutorial with iOS and Swift
- Advanced MapKit Tutorial: Custom Tiles
- MapKit Tutorial: Getting Started
Saving Data
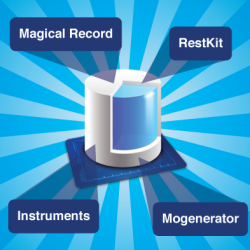
- Realm Tutorial: Getting Started
- SQLite Tutorial: Getting Started
- iOS Extensions: Document Provider Tutorial
- UIActivityViewController Tutorial: Sharing Data
- Getting Started with Core Data Tutorial
- Lightweight Migrations in Core Data Tutorial
- Multiple Managed Object Contexts with Core Data Tutorial
Networking
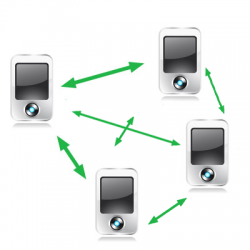
- Using NSURLProtocol with Swift
- User Authentication on iOS with Ruby on Rails and Swift
- Parse Tutorial: Getting Started with Web Backends
- OAuth 2.0 with Swift Tutorial
- CloudKit Tutorial: Getting Started
- CloudKit JS Tutorial for iOS
- Alamofire Tutorial: Getting Started
- Introduction to Protocol Buffers on iOS
- Real-Time Communication with Streams Tutorial for iOS
- URLSession Tutorial: Getting Started
Concurrency
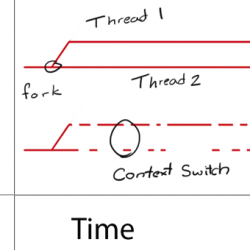
In these tutorials, you'll learn how to use concurrency the right way.
- Grand Central Dispatch Tutorial for Swift 3: Part 1/2
- Grand Central Dispatch Tutorial for Swift 3: Part 2/2
watchOS
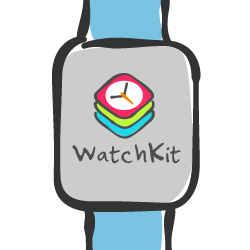
- WatchKit for watchOS 2: Initial Impressions
- watchOS 2 Tutorial Part 1: Getting Started
- watchOS 2 Tutorial Part 2: Tables
- watchOS 2 Tutorial Part 3: Animation
- watchOS 2 Tutorial Part 4: Watch Connectivity
- watchOS 3 Tutorial Part 1: Getting Started
- watchOS 3 Tutorial Part 2: Tables
- watchOS 3 Tutorial Part 3: Animation
Other Core APIs
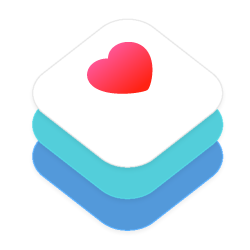
- EventKit Tutorial: Making a Calendar Reminder
- UIGestureRecognizer Tutorial: Getting Started
- Text Kit Tutorial: Getting Started
- Today Extensions Tutorial: Getting Started
- Handoff Tutorial: Getting Started
- NSRegularExpression Tutorial: Getting Started
- HealthKit Tutorial with Swift: Getting Started
- Apple Pay Tutorial: Getting Started
- HealthKit Tutorial with Swift: Workouts
- Address Book Tutorial in Swift and iOS
- ResearchKit Tutorial with Swift: Getting Started
- UIGestureRecognizer Tutorial: Creating Custom Recognizers
- iOS 9 Multitasking Tutorial
- iOS 9 App Search Tutorial: Introduction to App Search
- Accessing Heart Rate Data for Your ResearchKit Study
- In-App Purchase Tutorial: Getting Started
- JavaScriptCore Tutorial for iOS: Getting Started
- In App Purchases Tutorial: Consumables
- Universal Links – Make the Connection
- CareKit Tutorial for iOS: Part 1
- CareKit Tutorial for iOS: Part 2
- iOS Accessibility Tutorial: Getting Started
- Background Modes Tutorial: Getting Started
- In-App Purchases: Non-Renewing Subscriptions Tutorial
- CallKit Tutorial for iOS
- Today Extension Tutorial: Getting Started
- In-App Purchases: Auto-Renewable Subscriptions Tutorial
- SiriKit Tutorial for iOS
- Speech Recognition Tutorial for iOS
- Push Notifications Tutorial: Getting Started
- On-Demand Resources in iOS Tutorial
- Core ML and Vision: Machine Learning in iOS 11 Tutorial
Software Engineering
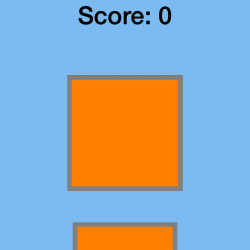
Here are a few tutorials on some of those - from an iOS-specific perspective!
- Intermediate Design Patterns in Swift
- Instruments Tutorial with Swift: Getting Started
- Creating and Distributing iOS Frameworks
- Intermediate Debugging with Xcode 8
Tools and Libraries
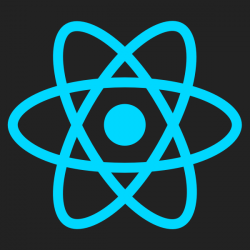
Here are some tutorials on some of the key tools and libraries you'll want to know about.
- Sponsored Tutorial: Improving Your App’s Performance with Pulse.io
- Facebook Tweaks with Swift Tutorial
- Google Maps iOS SDK Tutorial
- RubyMotion Tutorial for Beginners: Part 1
- RubyMotion Tutorial for Beginners: Part 2
- PaintCode Tutorial for Developers: Getting Started
- Facebook Shimmer Tutorial
- Tesseract OCR Tutorial
- Reveal Tutorial: Live View Debugging
- PaintCode Tutorial for Developers: Custom Progress Bar
- How to Create a CocoaPod in Swift
- PaintCode Tutorial for Designers: Getting Started
- Integrating Parse and React Native for iOS
- Getting Started with MagicalRecord
- Carthage Tutorial: Getting Started
- Travis CI Tutorial: Getting Started
- Firebase Tutorial: Getting Started
- Google Maps iOS SDK Tutorial: Getting Started
- What is… Nuke?
- AsyncDisplayKit 2.0 Tutorial: Getting Started
- AsyncDisplayKit 2.0 Tutorial: Automatic Layout
- How to Make App Mockups With AppCooker
- PaintCode Sketch Plugin Tutorial
- Core Plot Tutorial: Getting Started
- TestFlight Tutorial: iOS Beta Testing
- Building iOS Apps with Xamarin and Visual Studio
- Using Framer to Prototype iOS Animations
- React Native Tutorial: Integrating in an Existing App
- fastlane Tutorial: Getting Started
- Introduction to Google Cardboard for iOS
- Getting Started With RxSwift and RxCocoa
- Firebase Tutorial: Getting Started
- Firebase Tutorial: Real-time Chat
- Firebase Remote Config Tutorial for iOS
- WebSockets on iOS with Starscream
- Getting Started with PromiseKit
- AudioKit Tutorial: Getting Started
- Parse Server Tutorial with iOS
- IGListKit Tutorial: Better UICollectionViews
- Firebase Tutorial: iOS A/B Testing
- Bond Tutorial: Bindings in Swift
- Swift JSON Tutorial: Working with JSON
- How To Use Git Source Control with Xcode 8
- SwiftyBeaver Tutorial for iOS: A Logging Platform for Swift
- Charles Proxy Tutorial for iOS
- Dependency Management Using Git Submodules
- ReSwift Tutorial: Memory Game App
- Eureka Tutorial – Start Building Easy iOS Forms
- CocoaPods Tutorial for Swift: Getting Started
- Getting started with GraphQL & Apollo on iOS
- Sourcery Tutorial: Generating Swift code for iOS
- Yoga Tutorial: Using a Cross-Platform Layout Engine
- Assembly Register Calling Convention Tutorial
- Custom LLDB Commands in Practice
- React Native Tutorial: Building iOS and Android Apps with JavaScript
How-Tos
Readers frequently ask us for tutorials demonstrating how certain apps, animations, or controls are made. Your wish is our demand! :]Apps
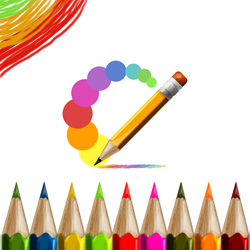
- How To Make a Gesture-Driven To-Do List App Like Clear in Swift: Part 1/2
- How To Make a Gesture-Driven To-Do List App Like Clear in Swift: Part 2/2
- How To Make a Letter / Word Game with UIKit and Swift: Part 1/3
- How To Make a Letter / Word Game with UIKit and Swift: Part 2/3
- How To Make a Letter / Word Game with UIKit and Swift: Part 3/3
- How To Make A Simple Drawing App with UIKit and Swift
- How To Make an App Like Runkeeper: Part 1
- How To Make an App Like Runkeeper: Part 2
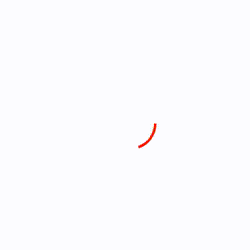
- How To Toss Views Using UIKit Dynamics
- How To Make a Table View Drop-In Card Animation
- How To Make A View Controller Transition Animation Like in the Ping App
- How To Create a Cool 3D Sidebar Animation Like in Taasky
- How To Implement A Circular Image Loader Animation with CAShapeLayer
- How to Create an iOS Book Open Animation: Part 1
- How to Create an iOS Book Open Animation: Part 2
- How To Create an Elastic Animation with Swift
- How to Create a Complex Loading Animation in Swift
- How To Create an Uber Splash Screen
- How To Make a Custom Control Tutorial: A Reusable Slider
- How to Create Your Own Slide-Out Navigation Panel in Swift
- How To Make a Custom Control Tutorial: A Reusable Knob
- How to Port Your App to the iPhone 6, iPhone 6 Plus and iOS 8: Top 10 Tips
- How To Secure Your App’s Passwords with Safari AutoFill in iOS 8
- How To Secure iOS User Data: The Keychain, Touch ID, and 1Password
- How To Create an Xcode Plugin: Part 1/3
- How To Create an Xcode Plugin: Part 2/3
- Swift Expanding Cells in iOS Collection Views
- How To Create an Xcode Plugin: Part 3/3
- How To Change Your App Icon at Build Time
- UICollectionView Custom Layout Tutorial: A Spinning Wheel
- How to Install iOS 9 and watchOS 2
- How to Submit An App to Apple: From No Account to App Store – Part 1
- How to Submit An App to Apple: From No Account to App Store – Part 2
Comments
Post a Comment